- Home /
Blit into Texture2DArray slice not working in WebGL
I am trying to copy the data from a texture into a RenderTexture set as array.
I wanted to use Graphics.CopyTexture but it isn't supported in WebGl so I fallback to using Graphics.Blit.
Blitting from a texture into the first slice of the Texture2DArray works fine, but all other indices only work in the editor and not on the WebGL build (using WebGL 2).
here is an image of the issue (left plane shows the source texture, right plane shows the texture array at slice 1)
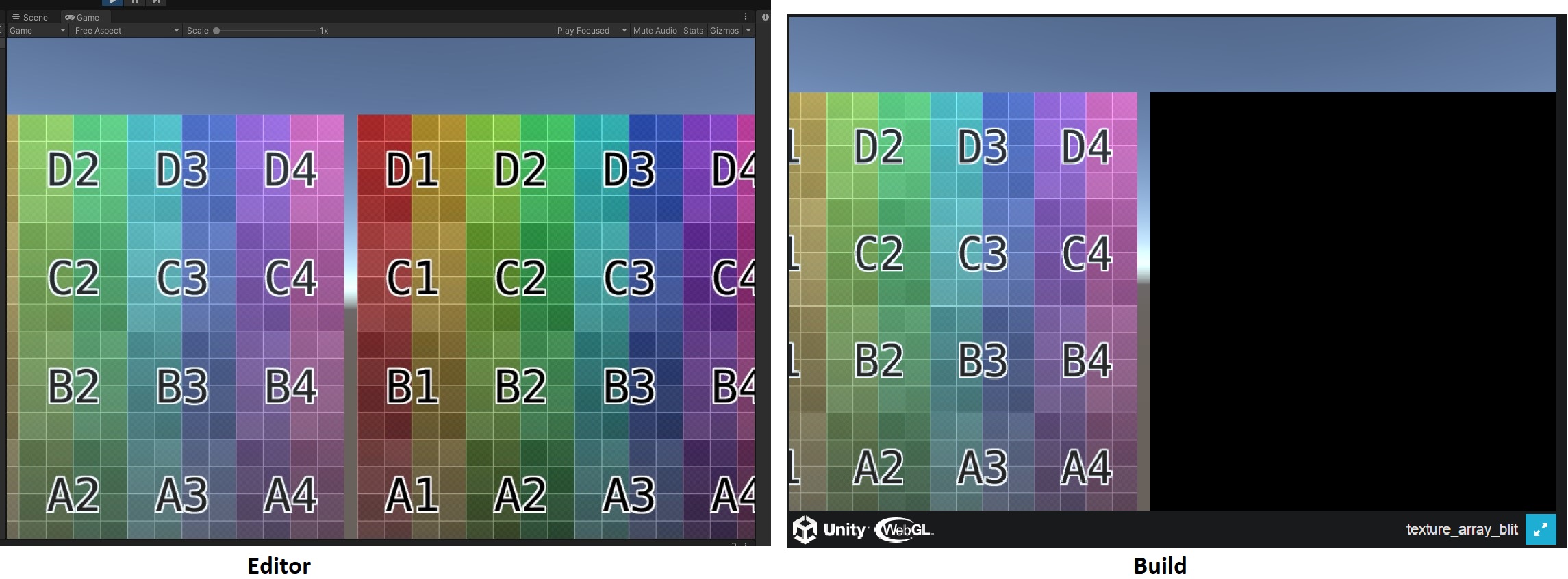
Here are the C# code and shader code:
C#
public class TextureArrayTest : MonoBehaviour
{
public Material textureArrayMat;
public Texture2D source;
public RenderTexture destination;
void Start()
{
destination = new RenderTexture(512, 512, 16);
destination.dimension = UnityEngine.Rendering.TextureDimension.Tex2DArray;
destination.volumeDepth = 2;
destination.Create();
textureArrayMat.SetTexture("_TextureAray", destination);
Graphics.Blit(source, destination, sourceDepthSlice: 0, destDepthSlice: 1);
}
}
Shader
Shader "Unlit/texture_array_test"
{
Properties
{
_TextureAray("Texture", 2DArray) = " " {}
}
SubShader
{
Tags { "RenderType"="Opaque" }
LOD 100
Pass
{
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#include "UnityCG.cginc"
struct appdata
{
float4 vertex : POSITION;
float2 uv : TEXCOORD0;
};
struct v2f
{
float2 uv : TEXCOORD0;
float4 vertex : SV_POSITION;
};
UNITY_DECLARE_TEX2DARRAY(_TextureAray);
v2f vert (appdata v)
{
v2f o;
o.vertex = UnityObjectToClipPos(v.vertex);
o.uv = v.uv;
return o;
}
fixed4 frag (v2f i) : SV_Target
{
fixed4 col = UNITY_SAMPLE_TEX2DARRAY(_TextureAray, float3(i.uv, 1));
return col;
}
ENDCG
}
}
}
Am doing something wrong or is this a bug ?
texture-results.jpg
(385.2 kB)
Comment
Your answer
