- Home /
Create a script that inherits from a class, and than attach multiple inherited classes to unity editor
Hello. So in the past I rarely if ever used inheritance and today I decided that this was probably the best case to use inheritance. So I don't actually know what to research to fix this :p.
What I've done so far
So basically I'm building an AI. The AI picks a goal to do. So in this case the goal is Food.I than want to create a List of Actions (The base class) for the goal, and the AI will follow these actions.
Actions are than made like GetFoodAction, and inherit from Actions and should be attached in the inspector.
The problem
Now the problem is that in unity the editor won't let me attach the actions. As you can see in the image below it has element 0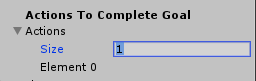
Here is the section that creates the list.
[Header("Actions To Complete Goal")]
public List<Actions> actions = new List<Actions>();
Here is the Actions Class
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[System.Serializable]
public class Actions
{
public virtual void Action()
{
}
}
Here is the overiding class
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GetFoodAction : Actions
{
public override void Action()
{
Debug.Log("Hello I will follow this action");
}
}
What I want
I need the element 0 to hold the GetFoodAction. Is this something I'm doing wrong? Or should I change my tactic of thinking?
Any help would be greatly appreciated.
Answer by Hellium · Nov 30, 2017 at 11:10 AM
I fell in love with Scriptable Objects recently, and I think it would be perfect in your case:
// Main script
[Header("Actions To Complete Goal")]
public List<Action> actions = new List<Action>();
// Action.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public abstract class Action : ScriptableObject
{
public abstract void Execute() ;
}
// GetFoodAction.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(fileName = "GetFoodAction", menuName = "Actions/GetFoodAction")]
public class GetFoodAction : Action
{
public override void Execute()
{
Debug.Log("I am hungry! Let's get some food!");
}
}
Then, in Unity, right click inside the "Project" Window, and click on "Create > Actions > GetFoodAction". A new asset will be created, and you will be able to drag & drop it in your list of actions.
That seems to do what I want thank you :p. I started have looked at scriptableObjects in the past but I never really used them and just kinda forgot about them. Thanks again
Yep, since they added the "CreateAsset$$anonymous$$enu" it has become really easy to use them.
However if the Action class actually contains state information it would be better to just inherit your Action class from $$anonymous$$onoBehaviour and attach the different Actions directly to your bot. That way every bot is carrying it's own instances around. That's actually the main purpose of a $$anonymous$$onoBehaviour, to specify the behaviour of the object.
ScriptableObjects are great when you just have pure functions (no state is stored in the class) and general readonly configuration data so the instances can be shared by all bots.
Answer by Thaun_ · Nov 30, 2017 at 11:01 AM
You cannot insert classes in a list directly, You either have to do
actions.Add(new Action());
Or create a gameobject, insert the script (oh wait it needs to have a monobehavior for that). And make it as a prefab, drag the object into the list and you are done. NOTE: You need to inherit MonoBehavior for this to work.
Your answer

Follow this Question
Related Questions
An OS design issue: File types associated with their appropriate programs 1 Answer
Multiple Cars not working 1 Answer
Accessing methods from another script. C# 1 Answer
Set default editor values for public variables in derived classes 0 Answers
I need to make two bullet controllers (friendly and enemy) Should I inherit? 1 Answer